Guidewire Gosu Tutorial
- Introduction to Guidewire Gosu
Guidewire is a powerful software suite widely used in the insurance industry for managing policies, claims, and billing operations. At the core of Guidewire applications lies Gosu, a statically-typed programming language that plays a crucial role in extending and customizing Guidewire products.
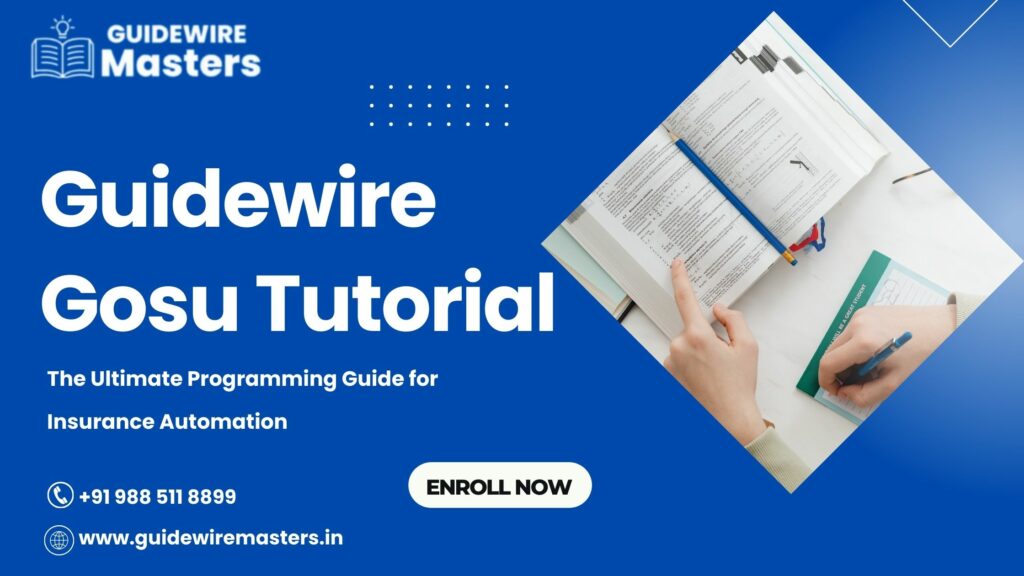
1. What is Gosu?
Gosu is a JVM-based programming language designed specifically for business applications. It shares similarities with Java but introduces dynamic typing, closures, and enhancements, making it more flexible for enterprise solutions. Developed by Guidewire, Gosu is the primary scripting language used to configure and extend PolicyCenter, BillingCenter, and ClaimCenter.
Importance of Gosu in the Guidewire Ecosystem
Gosu acts as the backbone of Guidewire applications. It allows developers to:
Customize business logic in PolicyCenter, BillingCenter, and ClaimCenter
Automate complex workflows in insurance operations
Create and extend functionalities using object-oriented programming
Since Guidewire is a market leader in insurance software, learning Gosu opens the door to numerous career opportunities.
2. Why Learn Gosu?
With the increasing adoption of Guidewire applications in the insurance sector, the demand for Gosu developers is rising. Let’s explore why learning Gosu is a valuable investment for IT professionals. in this Guidewire Gosu Tutorial Will Explain Everything why you to learn
Career Opportunities with Gosu Expertise
Companies using Guidewire actively seek developers skilled in Gosu. Here are some career benefits:
High-paying job opportunities in the insurance technology sector
Demand for Guidewire-certified professionals in major insurance firms
Versatility to work as a developer, consultant, or system integrator
How Gosu Improves Efficiency in Guidewire Applications
Gosu enhances efficiency by:
Enabling automation in insurance policy and claim processing
Providing a structured yet flexible programming approach
Reducing development time with built-in Guidewire APIs
By mastering Gosu, developers can build scalable and maintainable insurance solutions.
3. Understanding the Basics of Gosu
Before diving into coding, it’s essential to understand what makes Gosu unique.
History and Evolution of Gosu
Gosu originated as an internal scripting language for Guidewire applications. Over time, it evolved into a fully-fledged programming language with support for:
Static and dynamic typing
First-class functions
JVM interoperability
Differences Between Gosu and Java
Although Gosu runs on the JVM like Java, it has distinct advantages:
Enhancements: Unlike Java, Gosu allows adding methods to existing classes.
Closures: Gosu supports functional programming with closures and lambda expressions.
Better Type Inference: Gosu’s static and dynamic typing provide more flexibility than Java.
Understanding these differences helps developers transition from Java to Gosu seamlessly.
4. Setting Up the Development Environment
To start coding in Gosu, setting up a proper development environment is essential.
Required Software and Tools
Guidewire Studio: The official IDE for Gosu development.
JDK (Java Development Kit): Required to run Gosu programs.
Guidewire Sandbox: A testing environment for Guidewire applications.
Installing Guidewire Studio
Download the Guidewire Studio installer from the official Guidewire portal.
Follow the installation wizard to set up the environment.
Configure the necessary paths for Guidewire and Gosu development.
After installation, developers can start writing Gosu scripts within Guidewire applications.
5. Key Features of Gosu Programming Language
Gosu provides various features that make it a powerful language for enterprise applications. Find key feature of Gosu Programming Language in this Guidewire Gosu Tutorial.
Static and Dynamic Typing
Unlike traditional statically typed languages, Gosu supports dynamic typing, allowing developers to write flexible yet robust code.
Closures and Enhancements
Closures allow defining functions inline, making code more concise. Enhancements enable extending existing classes without modifying the original source code.
XML and JSON Processing
Gosu simplifies working with XML and JSON data, making it an excellent choice for API integrations.
With these capabilities, Gosu stands out as a practical and versatile language for insurance applications.
6. Data Types and Variables in Gosu
Understanding data types and variables is crucial for writing efficient Gosu programs. Gosu supports both primitive and complex data types, giving developers flexibility in handling data.
Primitive and Complex Data Types
Gosu provides various built-in data types, including:
Primitive Types: int, double, boolean, char
Complex Types: String, Date, List, Map
For example:
var age: int = 30
var name: String = “John Doe”
var isActive: boolean = true
Declaring and Initializing Variables
Variables in Gosu can be declared using var (for dynamic typing) or explicitly specifying the type.
var message = “Hello, Guidewire!” // Implicit type inference
var count: int = 5 // Explicit typing
Working with Lists and Maps
Lists and maps allow handling collections of data:
var numbers: List<Integer> = {1, 2, 3, 4, 5}
var userMap: Map<String, String> = {“name” -> “Alice”, “role” -> “Developer”}
7. Operators and Expressions in Gosu
Operators allow performing computations, comparisons, and logical operations in Gosu.
Arithmetic and Logical Operators
Gosu supports common arithmetic operators:
var sum = 10 + 5 // Addition
var difference = 10 – 5 // Subtraction
var product = 10 * 5 // Multiplication
var quotient = 10 / 5 // Division
var remainder = 10 % 3 // Modulus
Logical operators (&&, ||, !) are used for boolean expressions:
var isEligible = (age > 18) && (isActive)
String Concatenation
Strings can be concatenated using +:
var fullName = “John” + ” ” + “Doe”
Conditional Expressions
Ternary operators simplify conditional checks:
var status = (age > 18) ? “Adult” : “Minor”
8. Control Flow Statements in Gosu
Control flow statements allow executing code based on conditions and loops.
If-Else Statements
Conditional execution is handled using if-else:
if (age >= 18) {
print(“You are an adult.”)
} else {
print(“You are a minor.”)
}
Loops in Gosu
Gosu supports multiple looping constructs:
For Loop:
for (i in 1..5) {
print(i)
}
While Loop:
var count = 0
while (count < 5) {
print(count)
count++
}
while (count < 5) {
print(count)
count++
}
Do-While Loop:
gosu
var num = 0
do {
print(num)
num++
} while (num < 5)
Switch Statements in Gosu
<mark id=”p_15″>The switch statement is useful for handling multiple conditions:
gosu
var grade = “B”
switch (grade) {
case “A”: print(“Excellent”)
case “B”: print(“Good”)
case “C”: print(“Average”)
default: print(“Invalid Grade”)
}
Control flow statements improve decision-making and automation in Guidewire applications.
9. Functions and Methods in Gosu
Functions are reusable blocks of code that improve modularity.
Defining Functions
A simple function in Gosu looks like this:
gosu
function greet(name: String): String {
return “Hello, ” + name
}
print(greet(“Alice”))
Function Parameters and Return Types
Functions can take multiple parameters and return values:
go
function add(a: int, b: int): int {
return a + b
}
print(add(5, 10))
Function Overloading
Gosu supports function overloading, allowing multiple functions with the same name but different parameters:
gosu
function showMessage(message: String) {
print(message)
}
function showMessage(message: String, count: int) {
for (i in 1..count) {
print(message)
}
}
Functions make Gosu code reusable and efficient.
10. Object-Oriented Programming in Gosu
Gosu is an object-oriented language, supporting concepts like classes, objects, and inheritance.
Classes and Objects
A class in Gosu is defined using the class keyword:
gosu
class Person {
var name: String
var age: int
construct(name: String, age: int) {
this.name = name
this.age = age
}
function displayInfo() {
print(“Name: ” + name + “, Age: ” + age)
}
}
var person = new Person(“John Doe”, 30)
person.displayInfo()
Inheritance and Polymorphism
Classes can inherit from other classes:
gosu
class Employee extends Person {
var salary: double
construct(name: String, age: int, salary: double) {
super(name, age)
this.salary = salary
}
function showSalary() {
print(“Salary: ” + salary)
}
}
var emp = new Employee(“Alice”, 28, 50000)
emp.displayInfo()
emp.showSalary()
Encapsulation and Abstraction
Encapsulation restricts access to class members using access modifiers (private, public, protected).
Abstraction hides unnecessary details while exposing only essential functionalities.
Object-oriented programming in Gosu helps in structuring large-scale applications.
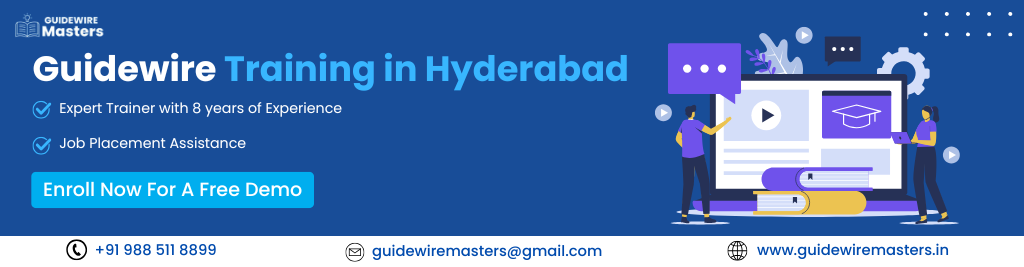
11. Gosu Enhancements and Extensions
Enhancements in Gosu allow adding new methods to existing classes without modifying their source code. This feature makes Gosu highly flexible and extensible. Do you Know Enchancements and Extensions in this Guidewire gosu Tutorial will explain Everything in this topic.
What Are Enhancements?
Enhancements provide a way to extend built-in types and third-party classes. Instead of modifying a class directly, you can create an enhancement that adds new methods or properties.
Creating and Using Enhancements
To define an enhancement, use the enhancement keyword:
gosu
enhancement StringEnhancement : String {
function reverse(): String {
return this.toCharArray().reverse().join(“”)
}
}
var text = “Guidewire”
print(text.reverse()) // Output: “eriwediuG”
Here, we’ve extended the String class by adding a reverse method.
Extending Existing Classes
Enhancements can also be applied to custom classes:
gosu
class Employee {
var name: String
construct(name: String) {
this.name = name
}
}
enhancement EmployeeEnhancement : Employee {
function greet() {
print(“Hello, ” + this.name)
}
}
var emp = new Employee(“Alice”)
emp.greet() // Output: “Hello, Alice”
Enhancements improve code reusability and reduce dependency on modifying core classes.
12. Exception Handling in Gosu
Error handling is an essential aspect of programming. Gosu provides robust mechanisms to handle exceptions gracefully.
Try-Catch-Finally Blocks
Gosu uses try-catch-finally blocks to manage exceptions:
gosu
try {
var num = 10 / 0 // Division by zero error
} catch (e: Exception) {
print(“An error occurred: ” + e.message)
} finally {
print(“Execution completed.”)
}
Custom Exceptions
You can define custom exceptions for specific scenarios:
gosu
class InvalidAgeException extends Exception {
construct(message: String) {
super(message)
}
}
function checkAge(age: int) {
if (age < 18) {
<mark id=”p_34″>throw new InvalidAgeException(“Age must be 18 or above.”)
}
print(“Valid age”)
}
try {
checkAge(16)
} catch (e: InvalidAgeException) {
print(e.message)
}
Best Practices for Error Handling
Use specific exception types instead of Exception for better clarity.
Log exceptions instead of just printing them.
Ensure finally blocks contain cleanup operations (e.g., closing database connections).
Effective error handling ensures application stability and reliability.
13. Working with XML and JSON in Gosu
Gosu simplifies working with XML and JSON data structures, making it easy to interact with APIs and data stores.
Parsing XML Data
XML data can be parsed using Gosu’s built-in support:
gosu
var xmlData = “<person><name>Alice</name><age>30</age></person>”
var xml = gw.xml.XmlElement.parse(xmlData)
print(xml.name) // Output: Alice
Working with JSON Objects
JSON is commonly used for data exchange. Gosu makes it easy to handle JSON:
gosu
var jsonData = ‘{“name”: “Alice”, “age”: 30}’
var json = gw.util.Json.parse(jsonData)
print(json.name) // Output: Alice
Serialization and Deserialization
Objects can be converted to and from JSON for easy storage and transmission:
gosu
class Person {
var name: String
var age: int
}
var person = new Person()
person.name = “Bob”
person.age = 25
var jsonString = gw.util.Json.serialize(person)
print(jsonString)
XML and JSON handling in Gosu enables seamless integration with web services and APIs.
14. Database Operations in Gosu
Gosu supports database connectivity, making it easy to run queries and manage records.
Connecting to a Database
Guidewire applications interact with databases using Gosu’s built-in persistence framework.
gosu
var db = gw.pl.persistence.core.Bundle
var result = db.query(“SELECT * FROM Person”)
print(result)
Running Queries with Gosu
You can retrieve records dynamically:
gosu
var people = gw.api.database.Query.make(Person).compare(“age”, “>”, 25).select()
for (person in people) {
print(person.name)
}
Handling Database Transactions
Transactions ensure data integrity during operations:
gosu
var bundle = gw.pl.persistence.core.Bundle.create()
var person = new Person()
person.name = “Charlie”
bundle.add(person)
bundle.commit() // Saves changes to the database
Database operations in Gosu help manage insurance policies, claims, and billing effectively.
15. Guidewire Integration with Gosu
Guidewire applications often need to integrate with external services via APIs. Gosu provides built-in support for web service integration.
Integrating with External APIs
Gosu can send and receive data from RESTful services:
gosu
var response = gw.util.Http.get(“https://api.example.com/users”)
print(response)
SOAP and RESTful Web Services
Gosu supports both SOAP and RESTful web services:
REST API Call:
gosu
var response = gw.util.Http.post(“https://api.example.com/data”, “{‘key’: ‘value’}”)
print(response)
SOAP Request:
gosu
var soapClient = gw.xml.soap.SoapClient.create(“https://example.com/service.wsdl”)
var result = soapClient.getService().fetchData()
print(result)
Data Exchange in Guidewire Applications
External system integrations allow Guidewire applications to:
Fetch insurance policy data from third-party providers
Process payment transactions securely
Automate claims verification with external databases
Guidewire integration ensures seamless communication between insurance software and external services.
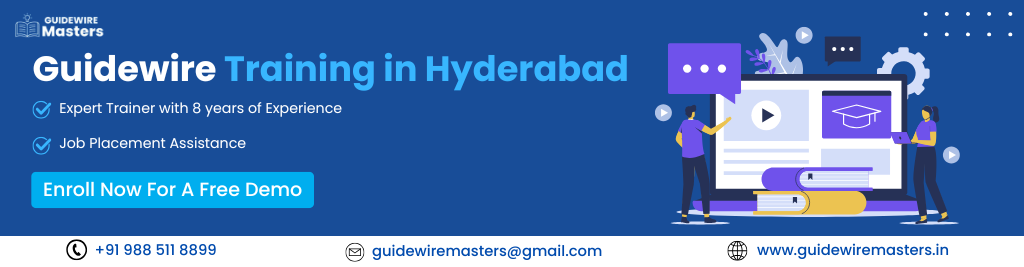
16. Unit Testing in Gosu
Testing is a critical part of software development. Gosu supports unit testing to ensure the reliability of applications.
Writing Test Cases in Gosu
Gosu provides a built-in testing framework to create unit tests:
gosu
uses gw.test.TestClass
@Test
function testAddition() {
var result = add(5, 3)
assertEquals(8, result)
}
Here, @Test defines a test case, and assertEquals checks if the function returns the expected value.
Mocking Dependencies
Mocking allows simulating dependencies for testing:
uses gw.mock.Mock
var mockService = Mock.create(MyService)
mockService.expect(“fetchData”).andReturn(“Mocked Data”)
This ensures tests run independently without requiring live services.
Running and Debugging Tests
To execute tests in Guidewire Studio:
Open the test class
Right-click and select Run Test
Check test results in the output console
Unit testing helps detect errors early and improves application stability.
17. Performance Optimization in Gosu
Optimizing Gosu code ensures better performance in Guidewire applications.
Best Practices for Writing Efficient Code
Minimize Loops: Avoid unnecessary loops to reduce execution time.
Use Caching: Store frequently accessed data to improve speed.
Optimize Database Queries: Fetch only required fields instead of retrieving entire records.
Avoiding Memory Leaks
Memory leaks can degrade performance. Best practices include:
Releasing unused objects
Avoiding circular references
Using weak references for caching
Profiling and Debugging Performance Issues
Guidewire Studio provides profiling tools to identify slow-running code. Use logging to detect bottlenecks:
gosu
uses gw.api.util.Logging
var logger = Logging.getLogger(“Performance”)
logger.info(“Execution started”)
Optimizing Gosu code enhances system responsiveness and user experience.
18. Security Best Practices in Gosu
Security is crucial for protecting sensitive data in insurance applications.
Handling Sensitive Data
Never store sensitive information in plain text. Use encryption:
gosu
uses gw.util.encryption.EncryptionUtil
var encryptedData = EncryptionUtil.encrypt(“password123”)
print(encryptedData)
Preventing Injection Attacks
SQL injection is a major security threat. Use parameterized queries instead of direct SQL execution:
gosu
var query = gw.api.database.Query.make(Person).compare(“name”, “=”, “Alice”).select()
Secure Coding Standards
Use role-based access control (RBAC) to restrict data access.
Keep Guidewire applications updated with security patches.
Implementing these practices ensures a secure Guidewire environment.
19. Real-World Use Cases of Gosu in Guidewire
Gosu plays a key role in automating insurance processes.
Practical Applications in Insurance
Policy Processing: Automating policy underwriting and issuance.
Claims Management: Validating and approving claims faster.
Billing Automation: Ensuring accurate premium calculations.
Automating Policy Processing
Gosu automates policy workflows:
gosu
function processPolicy(policy: Policy) {
if (policy.premium > 1000) {
policy.approve()
} else {
policy.reject()
}
}
Claims Management with Gosu
Gosu improves efficiency in claims processing:
gosu
function validateClaim(claim: Claim): boolean {
return claim.amount < 50000 && claim.isValid()
}
<mark id=”p_27″>These real-world applications highlight Gosu’s impact on the insurance industry.
20.Conclusion and Next Steps
Recap of Key Takeaways
Gosu is the core language for Guidewire applications.
It supports OOP, enhancements, and integrations.
Proper security and optimization enhance performance.
Further Learning Resources
To deepen your knowledge:
Refer to Guidewire documentation.
Take Gosu certification courses.
Join Guidewire developer communities.
Career Growth with Guidewire Gosu
Mastering Gosu opens doors to high-paying jobs in the insurance tech industry.
FAQS
1. Is Gosu similar to Java?
Yes, Gosu runs on the JVM and shares many similarities with Java but offers additional features like enhancements and better type inference.
2. Can I use Gosu outside of Guidewire?
Although primarily designed for Guidewire applications, Gosu can be used for standalone projects.
3. How do I start learning Gosu?
Start with Guidewire documentation, online courses, and practice within Guidewire Studio.
4. What companies hire Gosu developers?
Insurance companies, Guidewire partners, and IT consulting firms often hire Gosu experts.
5. Do I need prior programming experience to learn Gosu?
Basic knowledge of Java or OOP concepts can help, but beginners can learn Gosu with practice.
6. What is the main purpose of Gosu in Guidewire?
Gosu is used to extend and customize Guidewire applications, enabling developers to implement business logic, automate processes, and integrate external systems efficiently.
7. Is Gosu a strongly-typed language?
Yes, Gosu is statically typed, but it also supports dynamic typing, allowing developers to write flexible yet type-safe code.
8. How is Gosu different from Groovy?
While both are JVM-based languages, Gosu is statically typed and optimized for Guidewire applications, whereas Groovy is a dynamically-typed scripting language often used for general automation and scripting.
9. Can I use third-party Java libraries in Gosu?
Yes, since Gosu runs on the JVM, it allows seamless Java interoperability, meaning you can import and use Java libraries within your Gosu code
10. Does Gosu support functional programming?
Yes, Gosu supports closures (lambda expressions), allowing functional programming paradigms like passing functions as parameters and writing concise expressions.
11. What are Gosu Enhancements?
Enhancements allow developers to add new methods to existing classes without modifying their source code, making Gosu highly extensible.